C programming language is best known for offering various types of functionalities and features to the programmers. C also allows the programmers to do type casting.Typecasting and Type conversion are different things. In C, typecasting is a way to simply change the data type of a variable to another data type. Typecasting is so useful and efficient.
What is Type Casting in C ?
In C, When you convert the data type of a variable to another data type then this technique is known as typecasting.
Let’s say that you want to store a value of int data type into a variable of float data type.Then you can easily do this with the help of typecasting. It is one of the important concepts of the C programming language.
Syntax of Type Casting :
int num1; float num2; // BODY num2 = (float) num1; // Type Casting
Types of Type casting in C:-
There are two types of Type Casting in C-
- Implicit Type Casting
- Explicit Type Casting
1.Implicit Type Casting :-
Implicit type casting means conversion of data types without losing its original meaning. This type of typecasting is essential when you want to change data types without changing the significance of the values stored inside the variable.Implicit type conversion in C happens automatically when a value is copied to its compatible data type. During conversion, strict rules for type conversion are applied. If the operands are of two different data types, then an operand having lower data type is automatically converted into a higher data type. This type of type conversion can be seen in the following example.
#include <stdio.h> #include <stdlib.h> int main() { int a,b = 2; float x = 9.5; double y = 10.5; long int z = 50; double d; a = z/b+b*x-y; printf("When stored as integer : %d\n",a); d = z/b+b*x-y; printf("When stored as double : %f\n",d); return 0; }
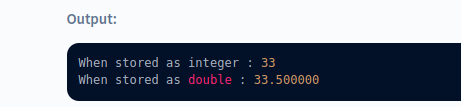
Important Points about Implicit Conversions
- Implicit type of type conversion is also called as standard type conversion. We do not require any keyword or special statements in implicit type casting.
- Converting from smaller data type into larger data type is also called as type promotion. In the above example, we can also say that the value of s is promoted to type integer.
- The implicit type conversion always happens with the compatible data types.
- We cannot perform implicit type casting on the data types which are not compatible with each other such as:
1. Converting float to an int will truncate the fraction part hence losing the meaning of the value.
2. Converting double to float will round up the digits.
3. Converting long int to int will cause dropping of excess high order bits.
In all the above cases, when we convert the data types, the value will lose its meaning. Generally, the loss of meaning of the value is warned by the compiler.‘C’ programming provides another way of typecasting which is explicit type casting.
2. Explicit Type Casting:-
Explicit type conversion is done by the user by using (type) operator. Before the conversion is performed, a runtime check is done to see if the destination type can hold the source value.
int a,c;
float b;
c = (int) a + b
Here, the resultant of ‘a+b’ is converted into ‘int’ explicitly and then assigned to ‘c’.
Examples of Explicit Type Casting :
#include<stdio.h> main(){ int i=40; short a; //Explicit conversion a=(short)i; printf("explicit value:%d\n",a); }
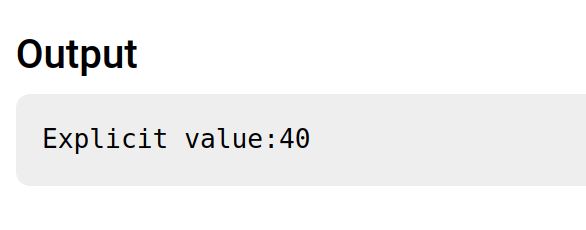