The goto statement is known as jump statement in C. As the namesuggests, goto is used to transfer the program control to a predefined
label. The goto statment can be used to repeat some part of the code fora particular condition. It can also be used to break the multiple loops which can’t be done by using a single break statement. However, using goto is avoided these days since it makes the program less readable and complecated.
Syntax of Go to Statement in C :
The label specified after the goto statement is the location where we place the code block to execute. From the below syntax, you can understand that we can place the label anywhere in the program. It doesn’t matter if you put before the goto or after.
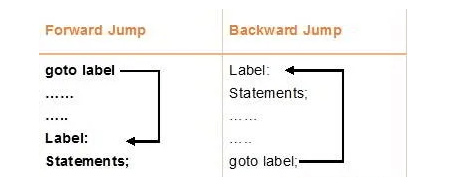
Flow Diagram of Go to Statement :
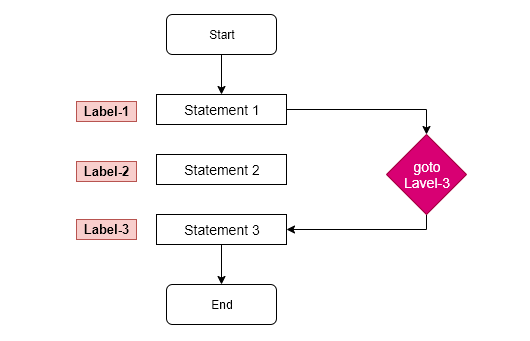
Examples :
Let’s see a simple example to use goto statement in C language.
#include <stdio.h> int main() { // we will print numbers from start to end // initialize start and end variables int start = 1, end = 10; // initialize variable to keep track of which number is to be printed int curr = start; // defining the label print_line : // print the current number printf("%d ", curr); // check if the current has reached the end // if not, that means some numbers are still to be printed if(curr<end) { // increment current curr++; // use goto to again repeat goto print_line; } // if the current has reached the end, the statements inside if will not be executed // the program terminates return 0; }
