Recursion is a process in which function call itself and the function that calls itself directly or indirectly called a recursive function. A recursive function calls itself so there can be several numbers of the recursive call, so the recursive function should have the termination condition to break the recursion. If there is a not termination condition in a recursive function, a stack overflow will occur and your program will crash.
Recursive functions are useful to solve many mathematical problems, like generating the Fibonacci series, calculating the factorial of a number, and convenient for recursively defined data structures like trees.
For example:
#include <stdio.h> int factorial(int number); int main() { int x = 6; printf("The factorial of %d is %d\n", x, factorial(x)); return 0;} int factorial(int number) { if (number == 1) return (1); /* exiting condition */ else return (number * factorial(number - 1)); }
Output
The factorial of 6 is 720
Here, we discuss program details:
- We declare our recursive factorial function which takes an integer parameter and returns the factorial of this parameter. This function will call itself and decrease the number until the exiting, or the base condition is reached. When the condition is true, the previously generated values will be multiplied by each other, and the final factorial value is returned.
- We declare and initialize an integer variable with value”6″ and then print its factorial value by calling our factorial function.
Consider the following chart to more understand the recursive mechanism which consists of calling the function its self until the base case or stopping condition is reached, and after that, we collect the previous values:
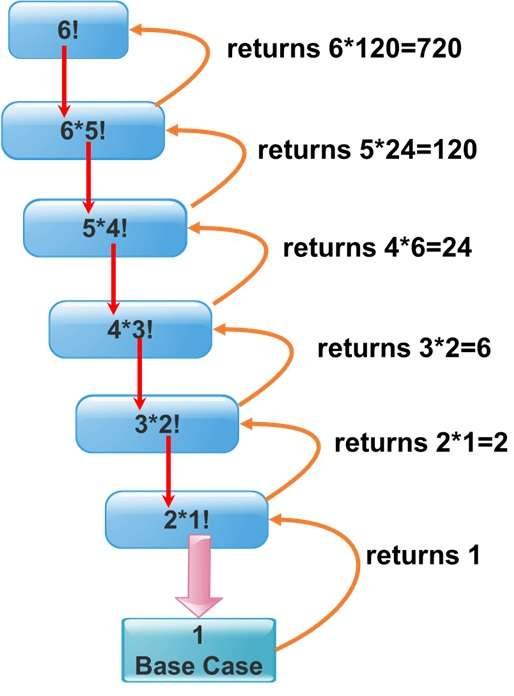