There are two methods to pass the data into the function in C language, i.e., call by value and call by reference.
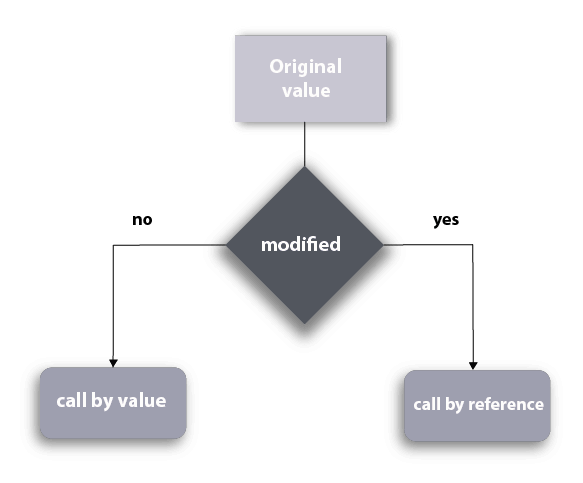
call by value
In the call by value, values of actual parameters are copied to function’s formal parameters and the two types of parameters are stored in different memory locations. So any changes made inside functions are not reflected in actual parameters of the caller.
I think now you are thinking about what is an actual parameter and formal parameters. So don’t worry I am explaining below about the actual and formal parameters.
Actual parameter : The value/variables which is passed by the caller while calling function. In simple words you can say, the parameters passed to a function.
Formal parameters : The identifier used in a function to stand for the value that is passed into the function by a caller. In simple words, the parameters received by a function.
Let’s see an example code:
#include <stdio.h> int sum(int a, int b) //Statement 1 { return a+b; } int main() { int x=10,y=20; int s = sum(x,y); //Statement 2 printf("%d\n",s); return 0; }
In Statement 1, the variables a and b are called FORMAL PARAMETERS. In Statement 2 the arguments x and y are called ACTUAL PARAMETERS (as they are the actual ones to hold the values)
So now comes on the topic, as I explained above that in the call by value any changes made inside functions are not reflected in actual parameters of the caller. The reason behind that a copy of each actual argument is assigned to the corresponding formal arguments. Let’s see an example code to understand this concept.
#include <stdio.h> void swap(int x, int y) { int c; c = x; x = y; y = c; printf("\nValue inside the function\n"); printf("x = %d , y = %d\n",x,y); } int main() { int x=10,y=20; printf("Initial value of x and y\n"); printf("x = %d , y = %d\n",x,y); printf("\n>>Calling the swap function\n"); swap(x,y); printf("\nValues after swap function call\n"); printf("x = %d , y = %d\n",x,y); return 0; }
Output:
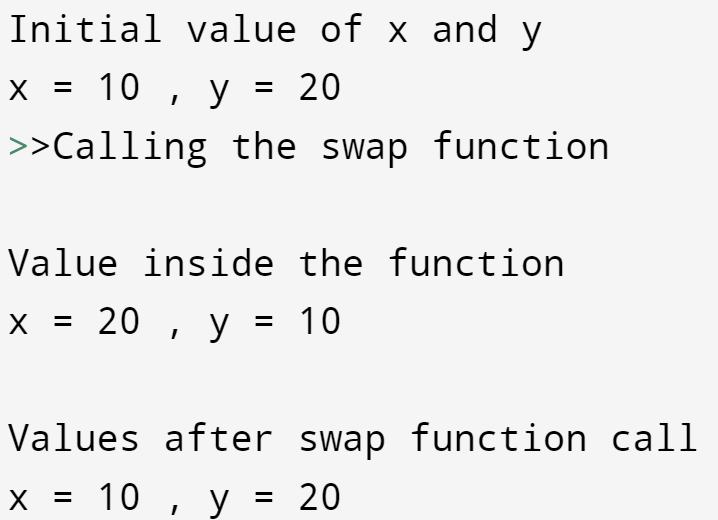
In the example code, swap() function modifies a copy of the values of x and y. However, the original value of x and y remains the same.
Call by Reference
In the call by reference, addresses of the actual arguments are copied and then assigned to the corresponding formal arguments. So in the call by reference both actual and formal parameters are pointing the same memory location. Therefore, any changes made to the formal parameters will get reflected in the actual parameters.
We have to remember two things before using this :
- We must pass the addresses of the actual arguments instead of passing values to the function.
- We must declare the formal arguments of the function as pointer variables of an appropriate type.
Note: Here I only explain call by reference in terms of C programming. In C++ we can use reference and pointer both for a call by reference.
Let’s see a C program to understand the call by reference,
#include <stdio.h> void swap(int *x, int *y) { int c; c = (*x); (*x) = (*y); (*y) = c; printf("\nValue inside the function\n"); printf("x = %d , y = %d\n",*x,*y); } int main() { int x=10,y=20; printf("Initial value of x and y\n"); printf("x = %d , y = %d\n",x,y); printf("\n>>Calling the swap function\n"); swap(&x,&y); printf("\nValues after swap function call\n"); printf("x = %d , y = %d\n",x,y); return 0; }
Output
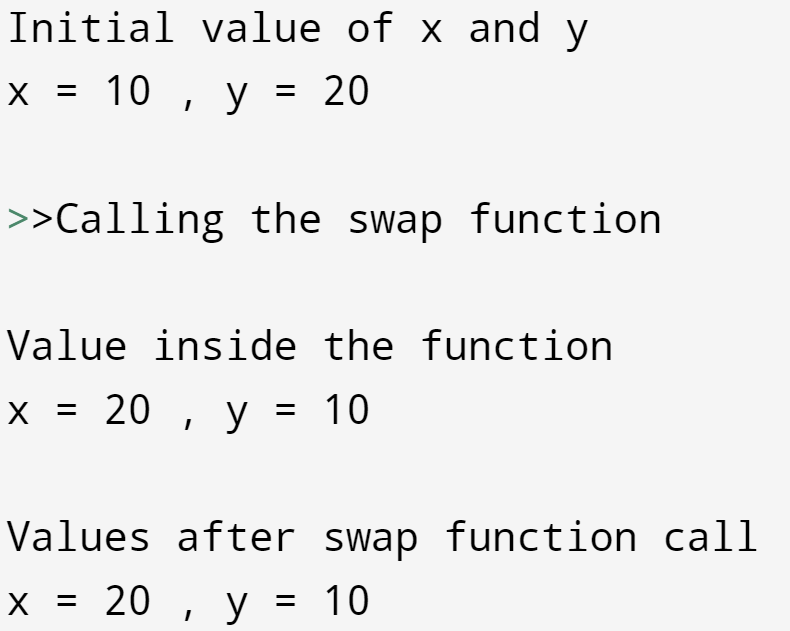
Explanation:
Here we are passing addresses of integer variables to a function. So the formal arguments must be declared as a pointer to int or (int *). Here formal and actual parameters point to the same memory location. So what we will change in formal parameters will reflect actual parameters.
c = (*x) =>> Means that first dereference the value at x then assigned to c (integer variable).
(x) = (y) =>> Means that first dereference the value at y then assigned to the address pointed by x.
(*y) = c =>> Means the value of c assigned to the address pointed by y
When the function swap() ends and control passes back to main() then still x and y contain the value which assigned in swap function. See the above-mentioned image.